通过Ajax动态加载MvcPager时,需要在包含MvcPager的PartialView中用Ajax.LoadMvcPagerScript();方法动态加载MvcPager客户端脚本库,而不是在主页面用Html.RegisterMvcPagerScriptResource();方法来注册。
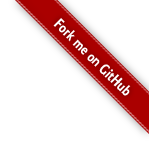
- Url路由分页
- Ajax分页
- Ajax搜索分页
- 外观样式
- 分页数据相关
- Javascrip API
- AjaxPager 类
- AjaxHelper.Pager 扩展方法
- HtmlPager 类
- HtmlHelper.Pager 扩展方法
- IPagedList 接口
- IPagedList<T> 接口
- MvcAjaxOptions 类
- MvcAjaxOptionsBuilder 类
- PagedList<T> 类
- PageLinqExtensions 类
-
PagerOptions 类
- ActionName属性
- AlwaysShowFirstLastPageNumber属性
- AutoHide属性
- ContainerTagName属性
- ControllerName属性
- CssClass属性
- CurrentPageNumberFormatString属性
- CurrentPagerItemTemplate属性
- DisabledPagerItemTemplate属性
- FirstPageRouteName属性
- FirstPageText属性
- GoToButtonId属性
- HidePagerItems属性
- HorizontalAlign属性
- HtmlAttributes属性
- Id属性
- InvalidPageIndexErrorMessage属性
- LastPageText属性
- MaximumPageIndexItems属性
- MaximumPageNumber属性
- MorePagerItemTemplate属性
- MorePageText属性
- NavigationPagerItemsPosition属性
- NavigationPagerItemTemplate属性
- NextPageText属性
- NumericPagerItemCount属性
- NumericPagerItemTemplate属性
- OnPageIndexError属性
- PageIndexBoxId属性
- PageIndexOutOfRangeErrorMessage属性
- PageIndexParameterName属性
- PageNumberFormatString属性
- PagerItemTemplate属性
- PrevPageText属性
- RouteName属性
- RouteValues属性
- ShowDisabledPagerItems属性
- ShowFirstLast属性
- ShowMorePagerItems属性
- ShowNumericPagerItems属性
- ShowPrevNext属性
- PagerItemsPosition 枚举
- PagerOptionsBuilder 类
- ScriptResourceExtensions 类
- Javascript API
- AjaxPager.cs
- AjaxPagerTest.cs
- DataLoaderBuilder.cs
- DisplayNameExtensions.cs
- HtmlPager.cs
- HtmlPagerTest.cs
- IPagedList.cs
- MvcAjaxOptions.cs
- MvcAjaxOptionsBuilder.cs
- MvcPager.js
- PagedList.cs
- PagedListTest.cs
- PageLinqExtensions.cs
- PagerBuilder.cs
- PagerExtensions.cs
- PagerItem.cs
- PagerItemsPosition.cs
- PagerOptions.cs
- PagerOptionsBuilder.cs
- ScriptResourceExtensions.cs
- TestHelper.cs
MvcPager 分页示例 — Ajax动态加载
该示例演示如何在需要时通过Ajax方法动态加载MvcPager和分页数据。
View:
@model PagedList<article> <button id="loadbtn">点此加载MvcPager和分页数据</button> <div id="articles"> </div> @section scripts { <script type="text/javascript"> $(function () { $("#loadbtn").click(function () { $("#articles").load(location.href); $(this).hide(); }); }); </script> }
_LoadByAjax.cshtml
@model PagedList<article> @{ Html.RenderPartial("_ArticleTable"); } @Ajax.Pager(Model, new PagerOptions { PageIndexParameterName = "id", ContainerTagName = "ul", PrevPageText = "上页", NextPageText = "下页", FirstPageText = "首页", LastPageText = "尾页", CssClass = "pagination", CurrentPagerItemTemplate = "<li class=\"active\"><a href=\"#\">{0}</a></li>",DisabledPagerItemTemplate = "<li class=\"disabled\"><a>{0}</a></li>",PagerItemTemplate = "<li>{0}</li>" }, new MvcAjaxOptions { UpdateTargetId = "articles" }) <script type="text/javascript"> $(function () { @{ Ajax.LoadMvcPagerScript(); } }); </script>
_ArticleTable.cshtml:
@model PagedList<Article> <table class="table table-bordered table-striped"> <tr> <th class="nowrap">序号</th> <th> @Html.DisplayNameFor(model => model.Title) </th> <th> @Html.DisplayNameFor(model => model.PubDate) </th> <th> @Html.DisplayNameFor(model => model.Author) </th> <th> @Html.DisplayNameFor(model => model.Source) </th> </tr> @{ int i = 0;} @foreach (var item in Model) { <tr> <td>@(Model.StartItemIndex + i++)</td> <td> @Html.DisplayFor(modelItem => item.Title) </td> <td> @Html.DisplayFor(modelItem => item.PubDate) </td> <td> @Html.DisplayFor(modelItem => item.Author) </td> <td> @Html.DisplayFor(modelItem => item.Source) </td> </tr> } </table>
Model:
public class Article { [Display(Name="文章编号")] public int ID { get; set; } [Display(Name="文章标题")] [MaxLength(200)] public string Title { get; set; } [Display(Name = "文章内容")] public string Content { get; set; } [Display(Name = "发布日期")] public DateTime PubDate { get; set; } [Display(Name = "作者")] [MaxLength(20)] public string Author { get; set; } [Display(Name = "文章来源")] [MaxLength(20)] public string Source { get; set; } }
Controller:
public ActionResult LoadByAjax(int id = 1) { using (var db = new DataContext()) { var model = db.Articles.OrderByDescending(a => a.PubDate).ToPagedList(id, 5); if (Request.IsAjaxRequest()) { return PartialView("_LoadByAjax", model); } return View(); } }